Victims
Victims are used to describe a specific organization or group that has been targeted or whose assets have been exploited.
Filtering Victims
This section provides the available filters which can be used when retrieving Victims from ThreatConnect.
Supported API Filters
API filters use the API filtering feature to limit the result set returned from the API.
Filter |
Value Type |
Description |
---|---|---|
|
int |
Filter Victims by ID. |
|
int |
Filter Victims on associated Adversary ID. |
|
int |
Filter Victims on associated Campaign ID. |
|
int |
Filter Victims on associated Document ID. |
|
int |
Filter Victims on associated Email ID. |
|
int |
Filter Victims on associated Incident ID. |
|
str |
Filter Victims on associated Indicator. |
|
list or str |
Filter Victims on Owner. |
|
str |
Filter Victims on applied Security Label. |
|
int |
Filter Victims on associated Signature ID. |
|
str |
Filter Victims on applied Tag. |
|
int |
Filter Victims on associated Task ID. |
|
int |
Filter Victims on associated Threat ID. |
Supported Post Filters
Post filters are applied on the results returned by the API request.
Filter |
Value Type |
Description |
---|---|---|
|
str |
Filter Victims on name. |
|
str |
Filter Victims on date added. |
Retrieve Victims
Retrieving a Single Victim
The example below demonstrates how to retrieve a specific Victim Resource from the ThreatConnect platform:
# replace the line below with the standard, TC script heading described here:
# https://docs.threatconnect.com/en/latest/python/quick_start.html#standard-script-heading
...
tc = ThreatConnect(api_access_id, api_secret_key, api_default_org, api_base_url)
# instantiate Victims object
victims = tc.victims()
owner = 'Example Community'
victim_id = 123456
# set a filter to retrieve the Victim with the id: 123456
filter1 = victims.add_filter()
filter1.add_owner(owner)
filter1.add_id(victim_id)
try:
# retrieve the Victim
victims.retrieve()
except RuntimeError as e:
print('Error: {0}'.format(e))
sys.exit(1)
try:
# prove there is only one Victim retrieved
assert len(victims) == 1
except AssertionError as e:
# if the Victim doesn't exist in the given owner, raise an error
print('AssertionError: The victim with ID {0} was not found in the "{1}" owner. '.format(victim_id, owner) +
'Try changing the `owner` variable to the name of an owner in your instance of ThreatConnect ' +
'and/or set the `victim_id` variable to the ID of a Victim that exists in the given owner.')
sys.exit(1)
# if the Victim was found, print some information about it
for victim in victims:
print(victim.id)
print(victim.name)
print(victim.nationality)
print(victim.org)
print(victim.suborg)
print(victim.work_location)
print(victim.weblink)
print('')
Note
If you get an AssertionError
when running this code, you likely need to change the name of the owner
variable so that it is the name of an owner in your instance of ThreatConnect and/or you need to change the victim_id
variable so that it is the ID of a Victim that exists in the given owner.
For details on how to retrieve victim assets, refer to the Victim Asset retrieval section.
Retrieving Multiple Victims
The example below demonstrates how to retrieve multiple Victim Resources from the ThreatConnect platform:
# replace the line below with the standard, TC script heading described here:
# https://docs.threatconnect.com/en/latest/python/quick_start.html#standard-script-heading
...
tc = ThreatConnect(api_access_id, api_secret_key, api_default_org, api_base_url)
# instantiate Victims object
victims = tc.victims()
owner = 'Example Community'
# set a filter to retrieve all Victims from the given owner that have the tag: 'Example'
filter1 = victims.add_filter()
filter1.add_owner(owner)
filter1.add_tag('Example')
try:
# retrieve the Victims
victims.retrieve()
except RuntimeError as e:
print('Error: {0}'.format(e))
sys.exit(1)
# iterate through the retrieved Victims and print their properties
for victim in victims:
print(victim.id)
print(victim.name)
print(victim.nationality)
print(victim.org)
print(victim.suborg)
print(victim.work_location)
print(victim.weblink)
print('')
Create Victims
The example below demonstrates how to create a Victim Resource in the ThreatConnect platform:
# replace the line below with the standard, TC script heading described here:
# https://docs.threatconnect.com/en/latest/python/quick_start.html#standard-script-heading
...
tc = ThreatConnect(api_access_id, api_secret_key, api_default_org, api_base_url)
# instantiate Victims object
victims = tc.victims()
owner = 'Example Community'
# create a new victim named 'Robin Scherbatsky' in the given owner
victim = victims.add('Robin Scherbatsky', owner)
try:
# create the Victim
victim.commit()
except RuntimeError as e:
print('Error: {0}'.format(e))
sys.exit(1)
Note
In the prior example, no API calls are made until the commit()
method is invoked.
Update Victims
The example below demonstrates how to update a Victim Resource in the ThreatConnect platform:
# replace the line below with the standard, TC script heading described here:
# https://docs.threatconnect.com/en/latest/python/quick_start.html#standard-script-heading
...
tc = ThreatConnect(api_access_id, api_secret_key, api_default_org, api_base_url)
# instantiate Victims object
victims = tc.victims()
owner = 'Example Community'
# create a Victim object with the name: 'Updated Victim'
victim = victims.add('Updated Victim', owner)
# set the id of the new Victim object to that of an existing Victim
victim.set_id(123456)
# you can update the Victim metadata as described here: https://docs.threatconnect.com/en/latest/python/victims/victims.html#victim-metadata
try:
# this will change the name of the Victim with id 123456 to: 'Updated Victim'
victim.commit()
except RuntimeError as e:
print('Error: {0}'.format(e))
sys.exit(1)
Note
In the prior example, no API calls are made until the commit()
method is invoked.
Delete Victims
The example below demonstrates how to delete a Victim Resource from the ThreatConnect platform:
# replace the line below with the standard, TC script heading described here:
# https://docs.threatconnect.com/en/latest/python/quick_start.html#standard-script-heading
...
tc = ThreatConnect(api_access_id, api_secret_key, api_default_org, api_base_url)
# instantiate Victims object
victims = tc.victims()
owner = 'Example Community'
# create an empty Victim object
victim = victims.add('', owner)
# set the id of the empty Victim object to the id of an existing Victim to delete
victim.set_id(123456)
try:
# delete the Victim
victim.delete()
except RuntimeError as e:
print(e)
sys.exit(1)
Note
In the prior example, no API calls are made until the delete()
method is invoked.
Victim Associations
Retrieve Victim Associations
The code snippet below demonstrates how to view Groups and Indicators which are associated with a given Victim in ThreatConnect. This example assumes there is a Victim with an ID of 123456
in the target owner. To test this code snippet, change the victim_id
variable.
Note
It is not possible to associate an Indicator directly with a Victim in ThreatConnect. The code below returns Indicators that share a Group association with the given Victim. In the image below, the Victim and Indicator are not directly associated, but are both associated with the same Group. Therefore, the Indicator would be returned when iterating through victim.indicator_associations
.
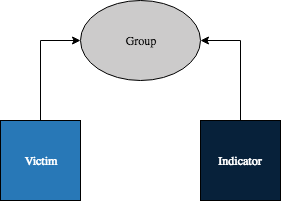
# replace the line below with the standard, TC script heading described here:
# https://docs.threatconnect.com/en/latest/python/quick_start.html#standard-script-heading
...
tc = ThreatConnect(api_access_id, api_secret_key, api_default_org, api_base_url)
# define the ID of the victim we would like to retrieve
victim_id = 123456
# create a victims object
victims = tc.victims()
# set a filter to retrieve the victim with the id: 123456
filter1 = victims.add_filter()
filter1.add_id(victim_id)
try:
# retrieve the Victims
victims.retrieve()
except RuntimeError as e:
print('Error: {0}'.format(e))
sys.exit(1)
# iterate through the Victims
for victim in victims:
print(victim.name)
# iterate through all associated groups
for associated_group in victim.group_associations:
# print details about the associated group
print(associated_group.id)
print(associated_group.name)
print(associated_group.resource_type)
print(associated_group.owner_name)
print(associated_group.date_added)
print(associated_group.weblink)
print('')
# iterate through all associated indicators
for associated_indicator in victim.indicator_associations:
# print details about the associated indicator
print(associated_indicator.id)
print(associated_indicator.indicator)
print(associated_indicator.type)
print(associated_indicator.description)
print(associated_indicator.owner_name)
print(associated_indicator.rating)
print(associated_indicator.confidence)
print(associated_indicator.date_added)
print(associated_indicator.last_modified)
print(associated_indicator.weblink)
print('')
Note
When the group_associations
and indicator_associations
properties are referenced, an API request is immediately invoked.
Create Victim Associations
The code snippet below demonstrates how to create an association between a Victim and another Group in ThreatConnect. This example assumes there is a Victim with an ID of 123456
and an Incident with an ID of 654321
in the target owner. To test this code snippet, change the victim_id
and incident_id
variables.
from threatconnect.Config.ResourceType import ResourceType
# replace the line below with the standard, TC script heading described here:
# https://docs.threatconnect.com/en/latest/python/quick_start.html#standard-script-heading
...
tc = ThreatConnect(api_access_id, api_secret_key, api_default_org, api_base_url)
# define the ID of the victim we would like to retrieve
victim_id = 123456
# define the ID of the incident we would like to associate with the victim
incident_id = 654321
# create a victims object
victims = tc.victims()
# set a filter to retrieve the victim with the id: 123456
filter1 = victims.add_filter()
filter1.add_id(victim_id)
try:
# retrieve the Victims
victims.retrieve()
except RuntimeError as e:
print('Error: {0}'.format(e))
sys.exit(1)
# iterate through the Victims
for victim in victims:
print(victim.name)
# create an association between this victim and the incident
victim.associate_group(ResourceType.INCIDENTS, incident_id)
# commit the changes to ThreatConnect
victim.commit()
Note
In the prior example, no API calls are made until the commit()
method is invoked.
Delete Victim Associations
The code snippet below demonstrates how to remove an association between a Victim and another Group. This example assumes there is a Victim with an ID of 123456
and an Incident with an ID of 654321
in the target owner. To test this code snippet, change the victim_id
and incident_id
variables.
from threatconnect.Config.ResourceType import ResourceType
# replace the line below with the standard, TC script heading described here:
# https://docs.threatconnect.com/en/latest/python/quick_start.html#standard-script-heading
...
tc = ThreatConnect(api_access_id, api_secret_key, api_default_org, api_base_url)
# define the ID of the victim we would like to retrieve
victim_id = 123456
# define the ID of the incident we would like to disassociate from the victim
incident_id = 654321
# create a victims object
victims = tc.victims()
# set a filter to retrieve the victim with the id: 123456
filter1 = victims.add_filter()
filter1.add_id(victim_id)
try:
# retrieve the Victims
victims.retrieve()
except RuntimeError as e:
print('Error: {0}'.format(e))
sys.exit(1)
# iterate through the Victims
for victim in victims:
print(victim.name)
# remove the association between this victim and the incident
victim.disassociate_group(ResourceType.INCIDENTS, incident_id)
# commit the changes to ThreatConnect
victim.commit()
Note
In the prior example, no API calls are made until the commit()
method is invoked.
Victim Metadata
Victim Attributes
Retrieve Victim Attributes
The code snippet below demonstrates how to retrieve the attributes from a Victim. This example assumes there is a Victim with an ID of 123456
in the target owner. To test this code snippet, change the victim_id
variable to the ID of a victim in your owner.
# replace the line below with the standard, TC script heading described here:
# https://docs.threatconnect.com/en/latest/python/quick_start.html#standard-script-heading
...
tc = ThreatConnect(api_access_id, api_secret_key, api_default_org, api_base_url)
# define the ID of the victim we would like to retrieve
victim_id = 123456
# create a victims object
victims = tc.victims()
# set a filter to retrieve the victim with the id: 123456
filter1 = victims.add_filter()
filter1.add_id(victim_id)
try:
# retrieve the Victims
victims.retrieve()
except RuntimeError as e:
print('Error: {0}'.format(e))
sys.exit(1)
# iterate through the Victims
for victim in victims:
print(victim.name)
# load the victim's attributes
victim.load_attributes()
for attribute in victim.attributes:
print(attribute.id)
print(attribute.type)
print(attribute.value)
print(attribute.date_added)
print(attribute.last_modified)
print(attribute.displayed)
print('')
Create Victim Attributes
The code snippet below demonstrates how to create an attribute on a Victim. This example assumes there is a Victim with an ID of 123456
in the target owner. To test this code snippet, change the victim_id
variable to the ID of a victim in your owner.
# replace the line below with the standard, TC script heading described here:
# https://docs.threatconnect.com/en/latest/python/quick_start.html#standard-script-heading
...
tc = ThreatConnect(api_access_id, api_secret_key, api_default_org, api_base_url)
# define the ID of the victim we would like to retrieve
victim_id = 123456
# create a victims object
victims = tc.victims()
# set a filter to retrieve the victim with the id: 123456
filter1 = victims.add_filter()
filter1.add_id(victim_id)
try:
# retrieve the Victims
victims.retrieve()
except RuntimeError as e:
print('Error: {0}'.format(e))
sys.exit(1)
# iterate through the Victims
for victim in victims:
print(victim.name)
# add a description attribute that is displayed at the top of the victim's page in ThreatConnect
victim.add_attribute('Description', 'Description Example', True)
# add a description attribute that is not displayed at the top of the victim's page in ThreatConnect
victim.add_attribute('Description', 'Description Example')
# commit the changes
victim.commit()
Note
In the prior example, no API calls are made until the commit()
method is invoked.
Update Victim Attributes
The code snippet below demonstrates how to update a Victim’s attribute. This example assumes there is a Victim with an ID of 123456
in the target owner. To test this code snippet, change the victim_id
variable to the ID of a victim in your owner.
# replace the line below with the standard, TC script heading described here:
# https://docs.threatconnect.com/en/latest/python/quick_start.html#standard-script-heading
...
tc = ThreatConnect(api_access_id, api_secret_key, api_default_org, api_base_url)
# define the ID of the victim we would like to retrieve
victim_id = 123456
# create a victims object
victims = tc.victims()
# set a filter to retrieve the victim with the id: 123456
filter1 = victims.add_filter()
filter1.add_id(victim_id)
try:
# retrieve the Victims
victims.retrieve()
except RuntimeError as e:
print('Error: {0}'.format(e))
sys.exit(1)
# iterate through the Victims
for victim in victims:
print(victim.name)
# load the victim's attributes
victim.load_attributes()
# iterate through the victim's attributes
for attribute in victim.attributes:
print(attribute.id)
# if the current attribute is a description attribute, update the value of the description
if attribute.type == 'Description':
victim.update_attribute(attribute.id, 'Updated Description')
# commit the changes
victim.commit()
Note
In the prior example, no API calls are made until the commit()
method is invoked.
Delete Victim Attributes
The code snippet below demonstrates how to delete a Victim’s attribute. This example assumes there is a Victim with an ID of 123456
in the target owner. To test this code snippet, change the victim_id
variable to the ID of a victim in your owner.
# replace the line below with the standard, TC script heading described here:
# https://docs.threatconnect.com/en/latest/python/quick_start.html#standard-script-heading
...
tc = ThreatConnect(api_access_id, api_secret_key, api_default_org, api_base_url)
# define the ID of the victim we would like to retrieve
victim_id = 123456
# create a victims object
victims = tc.victims()
# set a filter to retrieve the victim with the id: 123456
filter1 = victims.add_filter()
filter1.add_id(victim_id)
try:
# retrieve the Victims
victims.retrieve()
except RuntimeError as e:
print('Error: {0}'.format(e))
sys.exit(1)
# iterate through the Victims
for victim in victims:
print(victim.name)
# load the victim's attributes
victim.load_attributes()
# iterate through the victim's attributes
for attribute in victim.attributes:
print(attribute.id)
# if the current attribute is a description attribute, delete it
if attribute.type == 'Description':
victim.delete_attribute(attribute.id)
# commit the changes
victim.commit()
Note
In the prior example, no API calls are made until the commit()
method is invoked.
Victim Security Labels
Retrieve Victim Security Labels
The code snippet below demonstrates how to retrieve the security label from a Victim. This example assumes there is a Victim with an ID of 123456
in the target owner. To test this code snippet, change the victim_id
variable to the ID of a victim in your owner.
# replace the line below with the standard, TC script heading described here:
# https://docs.threatconnect.com/en/latest/python/quick_start.html#standard-script-heading
...
tc = ThreatConnect(api_access_id, api_secret_key, api_default_org, api_base_url)
# define the ID of the victim we would like to retrieve
victim_id = 123456
# create a victims object
victims = tc.victims()
# set a filter to retrieve the victim with the id: 123456
filter1 = victims.add_filter()
filter1.add_id(victim_id)
try:
# retrieve the Victims
victims.retrieve()
except RuntimeError as e:
print('Error: {0}'.format(e))
sys.exit(1)
# iterate through the Victims
for victim in victims:
print(victim.name)
# load the victim's security label
victim.load_security_label()
# if this victim has a security label, print some information about the sec. label
if victim.security_label is not None:
print(victim.security_label.name)
print(victim.security_label.description)
print(victim.security_label.date_added)
print('')
Warning
Currently, the ThreatConnect Python SDK does not support multiple security labels. If a Victim has multiple security labels, the Python SDK will only return one of them.
Create Victim Security Labels
The code snippet below demonstrates how to add a security label to a Victim. This example assumes there is a Victim with an ID of 123456
in the target owner. To test this code snippet, change the victim_id
variable to the ID of a victim in your owner.
# replace the line below with the standard, TC script heading described here:
# https://docs.threatconnect.com/en/latest/python/quick_start.html#standard-script-heading
...
tc = ThreatConnect(api_access_id, api_secret_key, api_default_org, api_base_url)
# define the ID of the victim we would like to retrieve
victim_id = 123456
# create a victims object
victims = tc.victims()
# set a filter to retrieve the victim with the id: 123456
filter1 = victims.add_filter()
filter1.add_id(victim_id)
try:
# retrieve the Victims
victims.retrieve()
except RuntimeError as e:
print('Error: {0}'.format(e))
sys.exit(1)
# iterate through the Victims
for victim in victims:
print(victim.name)
# add the 'TLP Green' label to the victim
victim.add_security_label('TLP Green')
# commit the victim with the new security label to ThreatConnect
victim.commit()
Note
In the prior example, no API calls are made until the commit()
method is invoked.
Delete Victim Security Labels
The code snippet below demonstrates how to delete a security label from a Victim. This example assumes there is a Victim with an ID of 123456
in the target owner. To test this code snippet, change the victim_id
variable to the ID of a victim in your owner.
# replace the line below with the standard, TC script heading described here:
# https://docs.threatconnect.com/en/latest/python/quick_start.html#standard-script-heading
...
tc = ThreatConnect(api_access_id, api_secret_key, api_default_org, api_base_url)
# define the ID of the victim we would like to retrieve
victim_id = 123456
# create a victims object
victims = tc.victims()
# set a filter to retrieve the victim with the id: 123456
filter1 = victims.add_filter()
filter1.add_id(victim_id)
try:
# retrieve the Victims
victims.retrieve()
except RuntimeError as e:
print('Error: {0}'.format(e))
sys.exit(1)
# iterate through the Victims
for victim in victims:
print(victim.name)
# remove the 'TLP Green' label from the victim
victim.delete_security_label('TLP Green')
# commit the victim with the removed security label to ThreatConnect
victim.commit()
Note
In the prior example, no API calls are made until the commit()
method is invoked.
Victim Assets
Victim Assets are accounts, infrastructure, and equipment that are used by the victim and targeted or exploited by an Adversary Threat. These include email accounts, social network accounts, network accounts, phones, or web sites. They can be associated to an incident, email, or threat as a targeted asset within ThreatConnect.
Retrieve Victim Assets
The example below demonstrates how to pull all Assets for the current Victim Resource from the ThreatConnect platform:
# replace the line below with the standard, TC script heading described here:
# https://docs.threatconnect.com/en/latest/python/quick_start.html#standard-script-heading
...
tc = ThreatConnect(api_access_id, api_secret_key, api_default_org, api_base_url)
# instantiate Victims object
victims = tc.victims()
# set a filter to retrieve the Victim with the id: 123456
filter1 = victims.add_filter()
filter1.add_id(123456)
try:
# retrieve the Victims
victims.retrieve()
except RuntimeError as e:
print('Error: {0}'.format(e))
sys.exit(1)
# iterate through the Victims
for victim in victims:
print(victim.id)
print(victim.name)
# retrieve the assets from ThreatConnect
victim.load_assets()
# iterate through the Victim's assets
for asset in victim.assets:
print(asset.id)
print(asset.name)
print(asset.type)
print(asset.weblink)
print('')
Create Victim Assets
The example below demonstrates how to create Victim Assets for an existing Victim with an ID of 123456
:
from threatconnect.Config.ResourceType import ResourceType
# replace the line below with the standard, TC script heading described here:
# https://docs.threatconnect.com/en/latest/python/quick_start.html#standard-script-heading
...
tc = ThreatConnect(api_access_id, api_secret_key, api_default_org, api_base_url)
# instantiate Victims object
victims = tc.victims()
# set a filter to retrieve the victim with the id: 123456
filter1 = victims.add_filter()
filter1.add_id(123456)
try:
# retrieve the Victims
victims.retrieve()
except RuntimeError as e:
print('Error: {0}'.format(e))
sys.exit(1)
# iterate through the Victims
for victim in victims:
# add email address asset to Victim
asset = VictimAssetObject(ResourceType.VICTIM_EMAIL_ADDRESSES)
asset.set_address('[email protected]')
asset.set_address_type('Personal')
victim.add_asset(asset)
# add network account asset to Victim
asset = VictimAssetObject(ResourceType.VICTIM_NETWORK_ACCOUNTS)
asset.set_account('victim')
asset.set_network('victimsareus Active Directory')
victim.add_asset(asset)
# add phone asset to Victim
asset = VictimAssetObject(ResourceType.VICTIM_PHONES)
asset.set_phone_type('555-555-5555')
victim.add_asset(asset)
# add social network asset to Victim
asset = VictimAssetObject(ResourceType.VICTIM_SOCIAL_NETWORKS)
asset.set_account('@victim')
asset.set_network('Twitter')
victim.add_asset(asset)
# add website asset to Victim
asset = VictimAssetObject(ResourceType.VICTIM_WEBSITES)
asset.set_website('www.example.com')
victim.add_asset(asset)
try:
# commit the Victim with the new Victim Assets
victim.commit()
except RuntimeError as e:
print('Error: {0}'.format(e))
sys.exit(1)
Note
In the prior example, no API calls are made until the commit()
method is invoked.
Update Victim Assets
The example below demonstrates how to update the Victim Assets of an existing Victim with an ID of 123456
:
from threatconnect.Config.ResourceType import ResourceType
# replace the line below with the standard, TC script heading described here:
# https://docs.threatconnect.com/en/latest/python/quick_start.html#standard-script-heading
...
tc = ThreatConnect(api_access_id, api_secret_key, api_default_org, api_base_url)
# instantiate Victims object
victims = tc.victims()
# set a filter to retrieve the Victim with the id: 123456
filter1 = victims.add_filter()
filter1.add_id(123456)
try:
# retrieve the Victims
victims.retrieve()
except RuntimeError as e:
print('Error: {0}'.format(e))
sys.exit(1)
# iterate through the Victims
for victim in victims:
print(victim.id)
print(victim.name)
# retrieve the assets from ThreatConnect
victim.load_assets()
# iterate through the Victim's assets
for asset in victim.assets:
# if the asset is an email address asset, update it
if asset.type == 'EmailAddress':
# create a new Victim asset object
new_asset = VictimAssetObject(ResourceType.VICTIM_EMAIL_ADDRESSES)
new_asset.set_address('[email protected]')
new_asset.set_address_type('Personal')
# update the existing asset with the new one
victim.update(asset.id, new_asset)
try:
# commit the Victim with the updated Victim Asset
victim.commit()
except RuntimeError as e:
print('Error: {0}'.format(e))
sys.exit(1)
Note
In the prior example, no API calls are made until the commit()
method is invoked.
Delete Victim Assets
The example below demonstrates how to delete Victim Assets from an existing Victim with an ID of 123456
:
# replace the line below with the standard, TC script heading described here:
# https://docs.threatconnect.com/en/latest/python/quick_start.html#standard-script-heading
...
tc = ThreatConnect(api_access_id, api_secret_key, api_default_org, api_base_url)
# instantiate Victims object
victims = tc.victims()
# set a filter to retrieve the Victim with the id: 123456
filter1 = victims.add_filter()
filter1.add_id(123456)
try:
# retrieve the Victims
victims.retrieve()
except RuntimeError as e:
print('Error: {0}'.format(e))
sys.exit(1)
# iterate through the Victims
for victim in victims:
print(victim.id)
print(victim.name)
# retrieve the assets from ThreatConnect
victim.load_assets()
# iterate through the Victim's assets
for asset in victim.assets:
# if the asset is a phone number asset, delete it
if asset.type == 'Phone':
victim.delete(asset.id, asset)
try:
# commit the Victim with the Victim Asset(s) deleted
victim.commit()
except RuntimeError as e:
print('Error: {0}'.format(e))
sys.exit(1)
Note
In the prior example, no API calls are made until the commit()
method is invoked.